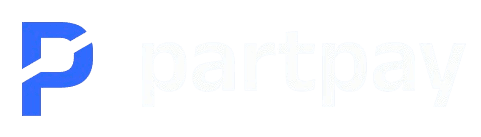
Create Vendor
Creating a New Vendor
The createVendor method creates a new vendor on the blockchain. It takes an object with the following properties:
- owner: UmiPublicKey of the vendor owner
- name: String name of the vendor
- metadata: Vendor object containing vendor details
Example usage
import { PartPayClient } from '@partpay/sdk';
import { Keypair } from '@solana/web3.js';
// The Solana RPC endpoint for communicating with the mainnet/devnet network.
const endpoint = const endpoint = 'https://api.devnet.solana.com';
// The secret (private) key for signing transactions.
const secretKey = Keypair.generate().secretKey;
// Initializes a PartPayClient using the specified Solana RPC endpoint and secret key for authentication.
const client = PartPayClient.createWithUmi(endpoint, secretKey);
const ownerPublicKey = Keypair.generate().publicKey;
const transactionBuilder = await client.createVendor({
owner: ownerPublicKey,
name: "My New Vendor",
image: "vendor image url, shop logo, etc"
metadata: {
shopName: "My Shop",
description: "A great place to buy equipment",
status: "active",
// add more vendor types
});
const signature = await client.sendAndConfirmTransaction(transactionBuilder);
console.log('Vendor created with signature:', signature);
} catch (error) {
console.error('Error creating vendor:', error);
}
This method returns a TransactionBuilder object. Use the sendAndConfirmTransaction method of the PartPayClient to execute the transaction on the blockchain.