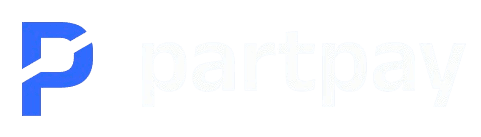
Update Equipment
Updating Equipment Metadata
The updateEquipment method updates the metadata of a specific piece of equipment. It takes an object with the following properties:
- equipmentPubKey: PublicKey of the equipment to be updated
- metadata: EquipmentMetadata object containing the updated equipment details
Example usage
import { PartPayClient } from '@partpay/sdk';
import { Keypair } from '@solana/web3.js';
// The Solana RPC endpoint for communicating with the mainnet/devnet network.
const endpoint = const endpoint = 'https://api.devnet.solana.com';
// The secret (private) key for signing transactions.
const secretKey = Keypair.generate().secretKey;
// Initializes a PartPayClient using the specified Solana RPC endpoint and secret key for authentication.
const client = PartPayClient.createWithUmi(endpoint, secretKey);
const equipmentPublicKey = Keypair.generate().publicKey;
const transactionBuilder = await client.updateEquipment({
equipmentPubKey: equipmentPublicKey,
metadata: {
name: "Updated Tractor",
description: "An updated powerful tractor",
price: 55000,
currency: "USDc",
stockQuantity: 2,
isActive: true,
// Include other required fields from EquipmentMetadata
}
});
const signature = await client.sendAndConfirmTransaction(transactionBuilder);
console.log('Equipment updated with signature:', signature);
This method returns a TransactionBuilder object. Use the sendAndConfirmTransaction method of the PartPayClient to execute the transaction on the blockchain.
Implementation Details
The updateEquipment method performs the following steps:
- Fetches the full asset information using the equipment's public key.
- Uploads the new metadata to obtain a new URI.
- Creates a transaction that updates the asset's metadata URI on the blockchain.