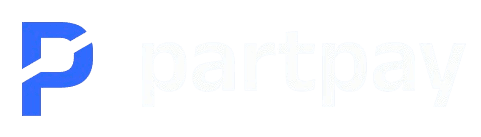
Set Financing Options
Setting Financing Options for Equipment
The setFinancingOptions method sets financing options for a specific piece of equipment. It takes an object with the following properties:
- equipment: UmiPublicKey of the equipment
- options: Array of FinancingOption objects
Example usage
import { PartPayClient } from '@partpay/sdk';
import { Keypair } from '@solana/web3.js';
async function setFinancingOptionsForEquipment() {
const endpoint = 'https://api.mainnet-beta.solana.com';
const secretKey = Keypair.generate().secretKey;
const client = PartPayClient.createWithUmi(endpoint, secretKey);
const equipmentPublicKey = Keypair.generate().publicKey;
try {
const transactionBuilder = await client.setFinancingOptions({
equipment: equipmentPublicKey,
options: [
{
term: 12,
termUnit: 'months',
interestRate: 5,
minimumDownPayment: BigInt(1000)
},
{
term: 24,
termUnit: 'months',
interestRate: 7,
minimumDownPayment: BigInt(500)
}
]
});
const signature = await client.sendAndConfirmTransaction(transactionBuilder);
console.log('Financing options set with signature:', signature);
} catch (error) {
console.error('Error setting financing options:', error);
}
}
setFinancingOptionsForEquipment();
This method returns a TransactionBuilder object. Use the sendAndConfirmTransaction method of the PartPayClient to execute the transaction on the blockchain.