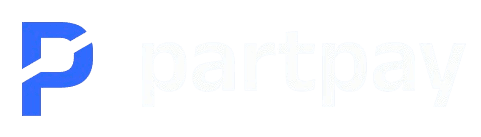
Add Equipment
Adding New Equipment
The addEquipment method adds a new piece of equipment to a vendor's inventory. It takes an object with the following properties:
- owner: UmiPublicKey of the equipment owner
- vendor: UmiPublicKey of the vendor
- name: String name of the equipment
- metadata: EquipmentMetadata object containing equipment details
Example usage
import { PartPayClient } from '@partpay/sdk';
import { Keypair } from '@solana/web3.js';
// The Solana RPC endpoint for communicating with the mainnet/devnet network.
const endpoint = const endpoint = 'https://api.devnet.solana.com';
// The secret (private) key for signing transactions.
const secretKey = Keypair.generate().secretKey;
// Initializes a PartPayClient using the specified Solana RPC endpoint and secret key for authentication.
const client = PartPayClient.createWithUmi(endpoint, secretKey);
const ownerPublicKey = Keypair.generate().publicKey;
const vendorPublicKey = Keypair.generate().publicKey;
const transactionBuilder = await client.addEquipment({
owner: ownerPublicKey,
vendor: vendorPublicKey,
name: "New Tractor",
metadata: {
description: "A powerful new tractor",
price: 50000,
currency: "USDC",
stockQuantity: 1,
isActive: true
}
});
const signature = await client.sendAndConfirmTransaction(transactionBuilder);
console.log('Equipment added with signature:', signature);
}
This method returns a TransactionBuilder object. Use the sendAndConfirmTransaction method of the PartPayClient to execute the transaction on the blockchain.