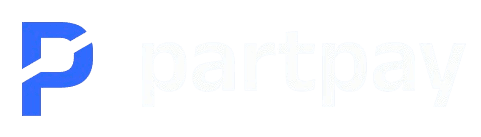
Create Installment Plan
Creating an Installment Plan
The createInstallmentPlan method creates an installment plan for financing equipment purchases. It takes an object with the following properties:
- equipment: UmiPublicKey of the equipment
- borrower: UmiPublicKey of the borrower
- totalAmount: Total amount to be financed
- installmentCount: Number of installments
- interestRate: Interest rate for the plan
- firstPaymentDate: Date of the first payment
- termUnit: 'days' | 'weeks' | 'months'
Example usage
import { PartPayClient } from '@partpay/sdk';
import { Keypair } from '@solana/web3.js';
async function createNewInstallmentPlan() {
const endpoint = 'https://api.mainnet-beta.solana.com';
const secretKey = Keypair.generate().secretKey;
const client = PartPayClient.createWithUmi(endpoint, secretKey);
const equipmentPublicKey = Keypair.generate().publicKey;
const borrowerPublicKey = Keypair.generate().publicKey;
try {
const transactionBuilder = await client.createInstallmentPlan({
equipment: equipmentPublicKey,
borrower: borrowerPublicKey,
totalAmount: 10000,
installmentCount: 12,
interestRate: 5,
firstPaymentDate: new Date('2023-07-01'),
termUnit: 'months'
});
const signature = await client.sendAndConfirmTransaction(transactionBuilder);
console.log('Installment plan created with signature:', signature);
} catch (error) {
console.error('Error creating installment plan:', error);
}
}
createNewInstallmentPlan();
This method returns a TransactionBuilder object. Use the sendAndConfirmTransaction method of the PartPayClient to execute the transaction on the blockchain.